Package Managers¶
nlohmann-json
nlohmann_json
nlohmann_json
nlohmann_json
nlohmann-json
Hunter nlohmann_json
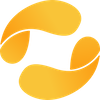
nlohmann-json
cget nlohmann/json
nlohmann/json
nlohmann.json
nlohmann_json
nlohmann-json
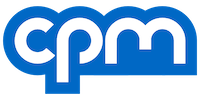
gh:nlohmann/json
nlohmann_json
Running example¶
Throughout this page, we will describe how to compile the example file example.cpp
below.
#include <nlohmann/json.hpp>
#include <iostream>
#include <iomanip>
using json = nlohmann::json;
int main()
{
std::cout << std::setw(4) << json::meta() << std::endl;
}
When executed, this program should create output similar to
{
"compiler": {
"c++": "201103",
"family": "gcc",
"version": "12.3.0"
},
"copyright": "(C) 2013-2022 Niels Lohmann",
"name": "JSON for Modern C++",
"platform": "apple",
"url": "https://github.com/nlohmann/json",
"version": {
"major": 3,
"minor": 11,
"patch": 3,
"string": "3.11.3"
}
}
Homebrew¶
Summary
formula: nlohmann-json
- Availalbe versions: current version and development version (with
--HEAD
parameter) - The formula is updated with every release.
- Maintainer: Niels Lohmann
- File issues at the Homebrew issue tracker
- Homebrew website
If you are using Homebrew, you can install the library with
brew install nlohmann-json
The header can be used directly in your code or via CMake.
Example: Raw compilation
-
Create the following file:
example.cpp#include <nlohmann/json.hpp> #include <iostream> #include <iomanip> using json = nlohmann::json; int main() { std::cout << std::setw(4) << json::meta() << std::endl; }
-
Install the package:
brew install nlohmann-json
-
Compile the code and pass the Homebrew prefix to the include path such that the library can be found:
c++ example.cpp -I$(brew --prefix nlohmann-json)/include -std=c++11 -o example
Example: CMake
-
Create the following files:
example.cpp#include <nlohmann/json.hpp> #include <iostream> #include <iomanip> using json = nlohmann::json; int main() { std::cout << std::setw(4) << json::meta() << std::endl; }
CMakeLists.txtcmake_minimum_required(VERSION 3.15) project(json_example) find_package(nlohmann_json CONFIG REQUIRED) add_executable(json_example example.cpp) target_link_libraries(json_example PRIVATE nlohmann_json::nlohmann_json)
-
Install the package:
brew install nlohmann-json
-
Compile the code and pass the Homebrew prefix to CMake to find installed packages via
find_package
:CMAKE_PREFIX_PATH=$(brew --prefix) cmake -S . -B build cmake --build build
Meson¶
Summary
wrap: nlohmann_json
- Availalbe versions: current version and select older versions (see WrapDB)
- The package is update automatically from file
meson.build
. - File issues at the library issue tracker
- Meson website
If you are using the Meson Build System, add this source tree as a meson subproject. You may also use the include.zip
published in this project's Releases to reduce the size of the vendored source tree. Alternatively, you can get a wrap file by downloading it from Meson WrapDB, or use
meson wrap install nlohmann_json
Please see the Meson project for any issues regarding the packaging.
The provided meson.build
can also be used as an alternative to CMake for installing nlohmann_json
system-wide in which case a pkg-config file is installed. To use it, have your build system require the nlohmann_json
pkg-config dependency. In Meson, it is preferred to use the dependency()
object with a subproject fallback, rather than using the subproject directly.
Example: Wrap
-
Create the following files:
meson.buildproject('json_example', 'cpp', version: '1.0', default_options: ['cpp_std=c++11'] ) dependency_json = dependency('nlohmann_json', required: true) executable('json_example', sources: ['example.cpp'], dependencies: [dependency_json], install: true )
example.cpp#include <nlohmann/json.hpp> #include <iostream> #include <iomanip> using json = nlohmann::json; int main() { std::cout << std::setw(4) << json::meta() << std::endl; }
-
Use the Meson WrapDB to fetch the nlohmann/json wrap:
mkdir subprojects meson wrap install nlohmann_json
-
Build:
meson setup build meson compile -C build
Bazel¶
Summary
use bazel_dep
, git_override
, or local_path_override
- Any version, that is available via Bazel Central Registry
- File issues at the library issue tracker
- Bazel website
This repository provides a Bazel MODULE.bazel
and a corresponding BUILD.bazel
file. Therefore, this repository can be referenced within a MODULE.bazel
by rules such as archive_override
, git_override
, or local_path_override
. To use the library you need to depend on the target @nlohmann_json//:json
(i.e., via deps
attribute).
Example
-
Create the following files:
BUILDcc_binary( name = "main", srcs = ["example.cpp"], deps = ["@nlohmann_json//:json"], )
WORKSPACEbazel_dep(name = "nlohmann_json", version = "3.11.3.bcr.1")
example.cpp#include <nlohmann/json.hpp> #include <iostream> #include <iomanip> using json = nlohmann::json; int main() { std::cout << std::setw(4) << json::meta() << std::endl; }
-
Build and run:
bazel build //:main bazel run //:main
Conan¶
Summary
recipe: nlohmann_json
- Availalbe versions: current version and older versions (see Conan Center)
- The package is update automatically via this recipe.
- File issues at the Conan Center issue tracker
- Conan website
If you are using Conan to manage your dependencies, merely add nlohmann_json/x.y.z
to your conanfile
's requires, where x.y.z
is the release version you want to use.
Example
-
Create the following files:
Conanfile.txt[requires] nlohmann_json/3.11.3 [generators] CMakeToolchain CMakeDeps
CMakeLists.txtcmake_minimum_required(VERSION 3.15) project(json_example) find_package(nlohmann_json REQUIRED) add_executable(json_example example.cpp) target_link_libraries(json_example PRIVATE nlohmann_json::nlohmann_json)
example.cpp#include <nlohmann/json.hpp> #include <iostream> #include <iomanip> using json = nlohmann::json; int main() { std::cout << std::setw(4) << json::meta() << std::endl; }
-
Call Conan:
conan install . --output-folder=build --build=missing
-
Build:
cmake -S . -B build -DCMAKE_TOOLCHAIN_FILE="conan_toolchain.cmake" -DCMAKE_BUILD_TYPE=Release cmake --build build
Spack¶
Summary
package: nlohmann-json
- Availalbe versions: current version and older versions (see Spack package)
- The package is updated with every release.
- Maintainer: Axel Huebl
- File issues at the Spack issue tracker
- Spack website
If you are using Spack to manage your dependencies, you can use the nlohmann-json
package via
spack install nlohmann-json
Please see the Spack project for any issues regarding the packaging.
Example
-
Create the following files:
CMakeLists.txtcmake_minimum_required(VERSION 3.15) project(json_example) find_package(nlohmann_json REQUIRED) add_executable(json_example example.cpp) target_link_libraries(json_example PRIVATE nlohmann_json::nlohmann_json)
example.cpp#include <nlohmann/json.hpp> #include <iostream> #include <iomanip> using json = nlohmann::json; int main() { std::cout << std::setw(4) << json::meta() << std::endl; }
-
Install the library:
spack install nlohmann-json
-
Load the environment for your Spack-installed packages:
spack load nlohmann-json
-
Build the project with CMake:
cmake -S . -B build -DCMAKE_PREFIX_PATH=$(spack location -i nlohmann-json) cmake --build build
Hunter¶
Summary
package: nlohmann_json
- Availalbe versions: current version and older versions (see Hunter package)
- The package is updated with every release.
- File issues at the Hunter issue tracker
- Hunter website
If you are using Hunter on your project for external dependencies, then you can use the nlohmann_json package via
hunter_add_package(nlohmann_json)
Please see the Hunter project for any issues regarding the packaging.
Example
-
Create the following files:
CMakeLists.txtcmake_minimum_required(VERSION 3.15) include("cmake/HunterGate.cmake") HunterGate( URL "https://github.com/cpp-pm/hunter/archive/v0.23.297.tar.gz" SHA1 "3319fe6a3b08090df7df98dee75134d68e2ef5a3" ) project(json_example) hunter_add_package(nlohmann_json) find_package(nlohmann_json CONFIG REQUIRED) add_executable(json_example example.cpp) target_link_libraries(json_example PRIVATE nlohmann_json::nlohmann_json)
example.cpp#include <nlohmann/json.hpp> #include <iostream> #include <iomanip> using json = nlohmann::json; int main() { std::cout << std::setw(4) << json::meta() << std::endl; }
-
Download required files
mkdir cmake wget https://raw.githubusercontent.com/cpp-pm/gate/master/cmake/HunterGate.cmake -O cmake/HunterGate.cmake
-
Build the project with CMake:
cmake -S . -B build cmake --build build
vcpkg¶
Summary
package: nlohmann-json
- Availalbe versions: current version
- The package is updated with every release.
- File issues at the vcpkg issue tracker
- vcpkg website
If you are using vcpkg on your project for external dependencies, then you can install the nlohmann-json package with
vcpkg install nlohmann-json
and follow the then displayed descriptions. Please see the vcpkg project for any issues regarding the packaging.
Example
-
Create the following files:
CMakeLists.txtcmake_minimum_required(VERSION 3.15) project(json_example) find_package(nlohmann_json CONFIG REQUIRED) add_executable(json_example example.cpp) target_link_libraries(json_example PRIVATE nlohmann_json::nlohmann_json)
example.cpp#include <nlohmann/json.hpp> #include <iostream> #include <iomanip> using json = nlohmann::json; int main() { std::cout << std::setw(4) << json::meta() << std::endl; }
-
Install package:
vcpkg install nlohmann-json
-
Build:
cmake -S . -B build -DCMAKE_TOOLCHAIN_FILE=$VCPKG_ROOT/scripts/buildsystems/vcpkg.cmake cmake --build build
cget¶
Summary
package: nlohmann/json
- Availalbe versions: current version and older versions
- The package is updated with every release.
- File issues at the cget issue tracker
- cget website
If you are using cget, you can install the latest master
version with
cget install nlohmann/json
A specific version can be installed with cget install nlohmann/json@v3.11.3
. Also, the multiple header version can be installed by adding the -DJSON_MultipleHeaders=ON
flag (i.e., cget install nlohmann/json -DJSON_MultipleHeaders=ON
).
Example
-
Create the following files:
CMakeLists.txtcmake_minimum_required(VERSION 3.15) project(json_example) find_package(nlohmann_json CONFIG REQUIRED) add_executable(json_example example.cpp) target_link_libraries(json_example PRIVATE nlohmann_json::nlohmann_json)
example.cpp#include <nlohmann/json.hpp> #include <iostream> #include <iomanip> using json = nlohmann::json; int main() { std::cout << std::setw(4) << json::meta() << std::endl; }
-
Initialize cget
cget init
-
Install the library
cget install nlohmann/json
-
Build
cmake -S . -B build -DCMAKE_TOOLCHAIN_FILE=cget/cget/cget.cmake cmake --build build
Swift Package Manager¶
Summary
package: nlohmann/json
- Availalbe versions: current version and older versions
- The package is updated with every release.
- File issues at the library issue tracker
- Xcode documentation
NuGet¶
Summary
package: nlohmann.json
- Availalbe versions: current and previous versions
- The package is updated with every release.
- Maintainer: Hani Kaabi
- File issues at the maintainer's issue tracker
- NuGet website
If you are using NuGet, you can use the package nlohmann.json with
dotnet add package nlohmann.json
Example
Probably the easiest way to use NuGet packages is through Visual Studio graphical interface. Just right-click on a project (any C++ project would do) in “Solution Explorer” and select “Manage NuGet Packages…”
Now you can click on “Browse” tab and find the package you like to install.
Most of the packages in NuGet gallery are .NET packages and would not be useful in a C++ project. Microsoft recommends adding “native” and “nativepackage” tags to C++ NuGet packages to distinguish them, but even adding “native” to search query would still show many .NET-only packages in the list.
Nevertheless, after finding the package you want, click on “Install” button and accept confirmation dialogs. After the package is successfully added to the projects, you should be able to build and execute the project without the need for making any more changes to build settings.
Note
A few notes:
- NuGet packages are installed per project and not system-wide. The header and binaries for the package are only available to the project it is added to, and not other projects (obviously unless we add the package to those projects as well)
- One of the many great things about your elegant work is that it is a header-only library, which makes deployment very straightforward. In case of libraries which need binary deployment (
.lib
,.dll
and.pdb
for debug info) the different binaries for each supported compiler version must be added to the NuGet package. Some library creators cram binary versions for all supported Visual C++ compiler versions in the same package, so a single package will support all compilers. Some others create a different package for each compiler version (and you usually see things like “v140” or “vc141” in package name to clarify which VC++ compiler this package supports). - Packages can have dependency to other packages, and in this case, NuGet will install all dependencies as well as the requested package recursively.
What happens behind the scenes
After you add a NuGet package, three changes occur in the project source directory. Of course, we could make these changes manually instead of using GUI:
-
A
packages.config
file will be created (or updated to include the package name if one such file already exists). This file contains a list of the packages required by this project (name and minimum version) and must be added to the project source code repository, so if you move the source code to a new machine, MSBuild/NuGet knows which packages it has to restore (which it does automatically before each build).<?xml version="1.0" encoding="utf-8"?> <packages> <package id="nlohmann.json" version="3.5.0" targetFramework="native" /> </packages>
-
A
packages
folder which contains actual files in the packages (these are header and binary files required for a successful build, plus a few metadata files). In case of this library for example, it containsjson.hpp
:Note
This directory should not be added to the project source code repository, as it will be restored before each build by MSBuild/NuGet. If you go ahead and delete this folder, then build the project again, it will magically re-appear!
-
Project MSBuild makefile (which for Visual C++ projects has a .vcxproj extension) will be updated to include settings from the package.
The important bit for us here is line 170, which tells MSBuild to import settings from
packages\nlohmann.json.3.5.0\build\native\nlohmann.json.targets
file. This is a file the package creator created and added to the package (you can see it is one of the two files I created in this repository, the other just contains package attributes like name and version number). What does it contain?For our header-only repository, the only setting we need is to add our include directory to the list of
AdditionalIncludeDirectories
:<?xml version="1.0" encoding="utf-8"?> <Project ToolsVersion="4.0" xmlns="http://schemas.microsoft.com/developer/msbuild/2003"> <ItemDefinitionGroup> <ClCompile> <AdditionalIncludeDirectories>$(MSBuildThisFileDirectory)include;%(AdditionalIncludeDirectories)</AdditionalIncludeDirectories> </ClCompile> </ItemDefinitionGroup> </Project>
For libraries with binary files, we will need to add
.lib
files to linker inputs and add settings to copy.dll
and other redistributable files to output directory, if needed.There are other changes to the makefile as well:
-
Lines 165-167 add the
packages.config
as one of project files (so it is shown in Solution Explorer tree view). It is added as None (no build action) and removing it wouldn’t affect build. -
Lines 172-177 check to ensure the required packages are present. This will display a build error if package directory is empty (for example when NuGet cannot restore packages because Internet connection is down). Again, if you omit this section, the only change in build would be a more cryptic error message if build fails.
Note
Changes to .vcxproj makefile should also be added to project source code repository.
-
As you can see, the mechanism NuGet uses to modify project settings is through MSBuild makefiles, so using NuGet with other build systems and compilers (like CMake) as a dependency manager is either impossible or more problematic than useful.
Please refer to this extensive description for more information.
Conda¶
Summary
package: nlohmann_json
- Availalbe versions: current and previous versions
- The package is updated with every release.
- File issues at the feedstock's issue tracker
- Conda documentation
If you are using conda, you can use the package nlohmann_json from conda-forge executing
conda install -c conda-forge nlohmann_json
Example
-
Create the following file:
example.cpp#include <nlohmann/json.hpp> #include <iostream> #include <iomanip> using json = nlohmann::json; int main() { std::cout << std::setw(4) << json::meta() << std::endl; }
-
Create and activate an anvironment "json`:
conda create -n json conda activate json
-
Install the package:
conda install -c conda-forge nlohmann_json
-
Build the code:
g++ -std=c++11 -I$(conda info --base)/envs/json/include example.cpp -o example
MSYS2¶
If you are using MSYS2, you can use the mingw-w64-nlohmann-json package, type pacman -S mingw-w64-i686-nlohmann-json
or pacman -S mingw-w64-x86_64-nlohmann-json
for installation. Please file issues here if you experience problems with the packages.
The package is updated automatically.
MacPorts¶
Summary
port: nlohmann-json
- Availalbe versions: current version
- The port is updated with every release.
- File issues at the MacPorts issue tracker
- MacPorts website
If you are using MacPorts, execute
sudo port install nlohmann-json
to install the nlohmann-json package.
Example: Raw compilation
-
Create the following file:
example.cpp#include <nlohmann/json.hpp> #include <iostream> #include <iomanip> using json = nlohmann::json; int main() { std::cout << std::setw(4) << json::meta() << std::endl; }
-
Install the package:
sudo port install nlohmann-json
-
Compile the code and pass the Homebrew prefix to the include path such that the library can be found:
c++ example.cpp -I/opt/local/include -std=c++11 -o example
Example: CMake
-
Create the following files:
example.cpp#include <nlohmann/json.hpp> #include <iostream> #include <iomanip> using json = nlohmann::json; int main() { std::cout << std::setw(4) << json::meta() << std::endl; }
CMakeLists.txtcmake_minimum_required(VERSION 3.15) project(json_example) find_package(nlohmann_json CONFIG REQUIRED) add_executable(json_example example.cpp) target_link_libraries(json_example PRIVATE nlohmann_json::nlohmann_json)
-
Install the package:
sudo port install nlohmann-json
-
Compile the code:
cmake -S . -B build cmake --build build
build2¶
If you are using build2
, you can use the nlohmann-json
package from the public repository http://cppget.org or directly from the package's sources repository. In your project's manifest
file, add depends: nlohmann-json
(probably with some version constraints). If you are not familiar with using dependencies in build2
, please read this introduction. Please file issues here if you experience problems with the packages.
The package is updated automatically.
bdep new -t exe -l c++
CPM.cmake¶
Summary
package: gh:nlohmann/json
- Availalbe versions: current and previous versions
- The package is updated with every release.
- File issues at the CPM.cmake issue tracker
- CPM.cmake website
If you are using CPM.cmake
, add the CPM.cmake script and the following snippet to your CMake project:
CPMAddPackage("gh:nlohmann/json@3.11.3")
Example
-
Create the following files:
example.cpp#include <nlohmann/json.hpp> #include <iostream> #include <iomanip> using json = nlohmann::json; int main() { std::cout << std::setw(4) << json::meta() << std::endl; }
CMakeLists.txtcmake_minimum_required(VERSION 3.15) project(json_example) include(${CMAKE_SOURCE_DIR}/cmake/CPM.cmake) CPMAddPackage("gh:nlohmann/json@3.11.3") add_executable(json_example example.cpp) target_link_libraries(json_example PRIVATE nlohmann_json::nlohmann_json)
-
Download CPM.cmake
mkdir -p cmake wget -O cmake/CPM.cmake https://github.com/cpm-cmake/CPM.cmake/releases/latest/download/get_cpm.cmake
-
Build
cmake -S . -B build cmake --build build
xmake¶
Summary
package: nlohmann_json
- Availalbe versions: current and previous versions
- The package is updated with every release.
- File issues at the xmake issue tracker
- xmake website
Example
-
Create the following files:
example.cpp#include <nlohmann/json.hpp> #include <iostream> #include <iomanip> using json = nlohmann::json; int main() { std::cout << std::setw(4) << json::meta() << std::endl; }
xmake.luaadd_requires("nlohmann_json") add_rules("mode.debug", "mode.release") target("xm") set_kind("binary") add_files("example.cpp") add_packages("nlohmann_json") set_languages("cxx11")
-
Build
xmake
-
Run
xmake run
Other package managers¶
The library is also contained in many other package repositories:
Buckaroo¶
If you are using Buckaroo, you can install this library's module with buckaroo add github.com/buckaroo-pm/nlohmann-json
. There is a demo repo here.
Warning
The module is outdated as the respective repository has not been updated in years.
CocoaPods¶
If you are using CocoaPods, you can use the library by adding pod "nlohmann_json", '~>3.1.2'
to your podfile (see an example). Please file issues here.
Warning
The module is outdated as the respective pod has not been updated in years.
wsjcpp¶
If you are using wsjcpp
, you can use the command wsjcpp install "https://github.com/nlohmann/json:develop"
to get the latest version. Note you can change the branch :develop
to an existing tag or another branch.
Warning
The package manager is outdated as the respective repository has not been updated in years.